垃圾评论太多,防不胜防,网上插件很多,但是闲着无聊自己写了一个!
实现的方式是手动添加IP到黑名单中,访客在提交评论或者通过post请求提交评论的时候,检查评论提交者的IP地址是否在黑名单中。如果IP地址在黑名单中,则拦截该评论,不允许其发表。
具体实现原理如下:
1、创建数据库表格: 在激活插件时,通过 comment_interceptor_create_table() 函数创建一个数据库表格,用于存储黑名单中的IP地址和备注信息。
2、添加管理页面: 使用 comment_interceptor_add_admin_page() 函数,在WordPress后台添加一个管理页面,用于管理IP地址的黑名单。
3、管理页面回调函数: 在管理页面回调函数 comment_interceptor_admin_page() 中,实现对IP地址黑名单的添加、删除和展示功能。用户可以在此页面通过表单添加新的IP地址到黑名单中,也可以删除已有的IP地址。
4、评论拦截: 使用 comment_interceptor_check_comment() 函数,对评论提交进行拦截检查。该函数会在评论提交前被调用,检查评论者的IP地址是否在黑名单中。如果在黑名单中,则会使用 wp_die() 函数中断评论提交流程,同时输出一条错误信息。
总体来说,这个插件提供了一个简单的IP地址黑名单管理功能,用于拦截在黑名单中的IP地址提交的评论,以提高WordPress站点的评论安全性。
PS:这个插件为了适配Puock的提示功能,最后使用了pk_comment_err函数,如果你是其他主题,请自行查找主题的错误提示函数!
<?php
/**
* Plugin Name: IP黑名单评论拦截
* Description: 自动拦截IP黑名单中地址提交的评论。
* Version: 1.2
* Author: 沛霖主页
* Author URI: https://ailcc.com
*/
// 创建表格
function comment_interceptor_create_table() {
global $wpdb;
$table_name = $wpdb->prefix . 'comment_interceptor';
$sql = "CREATE TABLE IF NOT EXISTS $table_name (
id INT(11) NOT NULL AUTO_INCREMENT,
ip_address VARCHAR(45) NOT NULL,
note TEXT NOT NULL,
PRIMARY KEY (id),
UNIQUE KEY ip_address (ip_address)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci";
require_once(ABSPATH . 'wp-admin/includes/upgrade.php');
dbDelta($sql);
}
register_activation_hook(__FILE__, 'comment_interceptor_create_table');
// 删除表格
function comment_interceptor_delete_table() {
global $wpdb;
$table_name = $wpdb->prefix . 'comment_interceptor';
$wpdb->query("DROP TABLE IF EXISTS $table_name");
}
register_deactivation_hook(__FILE__, 'comment_interceptor_delete_table');
// 添加管理页面
function comment_interceptor_add_admin_page() {
add_menu_page(
'IP黑名单管理',
'IP黑名单',
'manage_options',
'comment_interceptor',
'comment_interceptor_admin_page'
);
}
add_action('admin_menu', 'comment_interceptor_add_admin_page');
// 管理页面回调函数
function comment_interceptor_admin_page() {
global $wpdb;
$table_name = $wpdb->prefix . 'comment_interceptor';
// 处理表单提交
if (isset($_POST['submit']) && $_POST['submit'] === '添加') {
$ip_address = sanitize_text_field($_POST['ip_address']);
$note = sanitize_text_field($_POST['note']);
// 检查是否重复
$existing_record = $wpdb->get_row($wpdb->prepare("SELECT * FROM $table_name WHERE ip_address = %s", $ip_address));
if ($existing_record) {
echo '<div class="notice notice-error"><p>IP地址重复</p></div>';
} else {
$wpdb->insert(
$table_name,
array(
'ip_address' => $ip_address,
'note' => $note
),
array(
'%s',
'%s'
)
);
echo '<div class="notice notice-success"><p>IP地址添加成功。</p></div>';
}
}
// 处理删除操作
if (isset($_GET['action']) && $_GET['action'] === 'delete' && isset($_GET['id'])) {
$id = intval($_GET['id']);
$wpdb->delete(
$table_name,
array('id' => $id),
array('%d')
);
echo '<div class="notice notice-success"><p>IP地址删除成功。</p></div>';
}
// 显示 IP 记录列表
$ip_records = $wpdb->get_results("SELECT * FROM $table_name");
?>
<div class="wrap">
<h1>评论拦截管理</h1>
<h2>IP地址记录</h2>
<table class="wp-list-table widefat fixed striped">
<thead>
<tr>
<th>ID</th>
<th>IP地址</th>
<th>备注</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<?php foreach ($ip_records as $record) : ?>
<tr>
<td><?php echo $record->id; ?></td>
<td><?php echo $record->ip_address; ?></td>
<td><?php echo $record->note; ?></td>
<td>
<a href="?page=comment_interceptor&action=delete&id=<?php echo $record->id; ?>">删除</a>
</td>
</tr>
<?php endforeach; ?>
</tbody>
</table>
<h2>添加IP地址</h2>
<form method="post">
<table class="form-table">
<tr>
<th scope="row"><label for="ip_address">IP地址</label></th>
<td><input type="text" name="ip_address" id="ip_address" required></td>
</tr>
<tr>
<th scope="row"><label for="note">备注</label></th>
<td><input type="text" name="note" id="note"></td>
</tr>
</table>
<p class="submit">
<input type="submit" name="submit" id="submit" class="button button-primary" value="添加">
</p>
</form>
</div>
<?php
}
// 拦截评论
function comment_interceptor_check_comment($commentdata) {
global $wpdb;
$table_name = $wpdb->prefix . 'comment_interceptor';
$ip_address = isset($_SERVER['HTTP_X_FORWARDED_FOR']) ? sanitize_text_field($_SERVER['HTTP_X_FORWARDED_FOR']) : sanitize_text_field($_SERVER['REMOTE_ADDR']);
// 检查是否存在记录
$existing_record = $wpdb->get_row($wpdb->prepare("SELECT * FROM $table_name WHERE ip_address = %s", $ip_address));
if ($existing_record) {
pk_comment_err('您的IP地址已被拦截,无法发表评论!');
wp_die();
}
return $commentdata;
}
add_filter('preprocess_comment', 'comment_interceptor_check_comment');
共计31人点赞,其中5人来自小程序
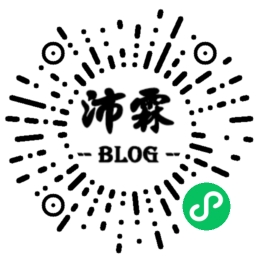